Before implement twitter login authentication we need to get consumerKey and consumerSecret key from twitter for that we need to create application in twitter by using this linkhttps://dev.twitter.com/apps/new once open that will display window like this
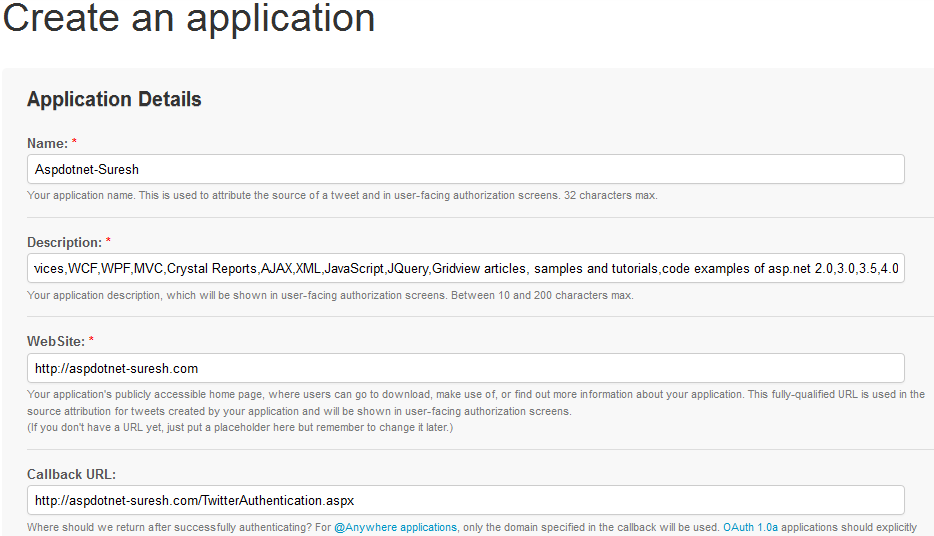
<html xmlns="http://www.w3.org/1999/xhtml" >
<head id="Head1">
<title>Twitter Login Authentication for Website in asp.net</title>
</head>
<body>
<form id="form1" runat="server">
<asp:ImageButton ID="imgTwitter" runat="server" ImageUrl="~/TwitterSigning.png"
onclick="imgTwitter_Click" />
<table id="tbleTwitInfo" runat="server" border="1" cellpadding="4" cellspacing="0" visible="false">
<tr>
<td colspan="2"><b>Twitter User Profile</b></td>
</tr>
<tr>
<td><b>UserName:</b></td>
<td><%=username%></td>
</tr>
<tr>
<td><b>Full Name:</b></td>
<td><%=name%></td>
</tr>
<tr>
<td><b>Profile Image:</b></td>
<td><img src="<%=profileImage%>" /></td>
</tr>
<tr>
<td><b>Twitter Followers:</b></td>
<td><%=followersCount%></td>
</tr>
<tr>
<td><b>Number Of Tweets:</b></td>
<td><%=noOfTweets%></td>
</tr>
<tr>
<td><b>Recent Tweet:</b></td>
<td><%=recentTweet%></td>
</tr>
</table>
</form>
</body>
</html>
|
Now in code behind add following namespaces
C# Code
C# Code
using System;
using System.Web.UI;
using System.Xml;
using oAuthExample;
|
After completion of adding namespaces write following code in code behind
string url = "";
string xml = "";
public string name = "";
public string username = "";
public string profileImage = "";
public string followersCount = "";
public string noOfTweets = "";
public string recentTweet = "";
protected void Page_Load(object sender, EventArgs e)
{
GetUserDetailsFromTwitter();
}
private void GetUserDetailsFromTwitter()
{
if(Request["oauth_token"]!=null & Request["oauth_verifier"]!=null)
{
imgTwitter.Visible = false;
tbleTwitInfo.Visible = true;
var oAuth = new oAuthTwitter();
//Get the access token and secret.
oAuth.AccessTokenGet(Request["oauth_token"], Request["oauth_verifier"]);
if (oAuth.TokenSecret.Length > 0)
{
//We now have the credentials, so make a call to the Twitter API.
url = "http://twitter.com/account/verify_credentials.xml";
xml = oAuth.oAuthWebRequest(oAuthTwitter.Method.GET, url, String.Empty);
XmlDocument xmldoc=new XmlDocument();
xmldoc.LoadXml(xml);
XmlNodeList xmlList = xmldoc.SelectNodes("/user");
foreach (XmlNode node in xmlList)
{
name = node["name"].InnerText;
username = node["screen_name"].InnerText;
profileImage = node["profile_image_url"].InnerText;
followersCount = node["followers_count"].InnerText;
noOfTweets = node["statuses_count"].InnerText;
recentTweet = node["status"]["text"].InnerText;
}
}
}
}
protected void imgTwitter_Click(object sender, ImageClickEventArgs e)
{
var oAuth = new oAuthTwitter();
if (Request["oauth_token"] == null)
{
//Redirect the user to Twitter for authorization.
//Using oauth_callback for local testing.
oAuth.CallBackUrl = "http://aspdotnet-suresh.com/TwitterAuthentication.aspx";
Response.Redirect(oAuth.AuthorizationLinkGet());
}
else
{
GetUserDetailsFromTwitter();
}
}
|
If you observe above code I used oAuthTwitter class file you can get this class file from downloadable code. Now get consumerKey and consumerSecret key from twitter and add it in web.config file like this
<appSettings>
<add key="consumerKey" value="Gyew474of7tpEBqnpDw" />
<add key="consumerSecret" value="ytgklq3b8lkxgPShCWeawqzrYpUa1bgsaeGRwW" />
</appSettings>
![]() |
No comments:
Post a Comment